35.1 Introduction
Error handling is an essential procedure in Visual Basic programming because it can help make the program error-free. An error-free program can run smoothly and efficiently, and the user does not have to face all sorts of problems such as program crash or system hang.
Errors often occur due to incorrect input from the user. For example, the user might make the mistake of attempting to ask the computer to divide a number by zero which will definitely cause system error. Another example is the user might enter a text (string) to a box that is designed to handle only numeric values such as the weight of a person, the computer will not be able to perform arithmetic calculation for text therefore will create an error. These errors are known as synchronous errors.
Therefore a good programmer should be more alert to the parts of program that could trigger errors and should write errors handling code to help the user in managing the errors. Writing errors handling code should be considered a good practice for Visual Basic programmers, so do try to finish a program fast by omitting the errors handling code. However, there should not be too many errors handling code in the program as it create problems for the programmer to maintain and troubleshoot the program later.
35.2 Writing the Errors Handling Code
We shall now learn how to write errors handling code in Visual Basic. The syntax for errors handling is
On Error GoTo program_label
where program_label is the section of code that is designed by the programmer to handle the error committed by the user. Once an error is detected, the program will jump to the program_label section for error handling.
Example 35.1: Division by Zero
Private Sub CmdCalculate_Click()Dim firstNum, secondNum As Double
firstNum = Txt_FirstNumber.Text
secondNum = Txt_SecondNumber.Text
On Error GoTo error_handler
Lbl_Answer.Caption = firstNum / secondNum
Exit Sub 'To prevent error handling even the inputs are valid
error_handler:
Lbl_Answer.Caption = "Error"
Lbl_ErrorMsg.Visible = True
Lbl_ErrorMsg.Caption = " You attempt to divide a number by zero!Try again!"End Sub
Private Sub Txt_FirstNumber_GotFocus()Lbl_ErrorMsg.Visible = FalseEnd SubPrivate Sub Txt_SecondNumber_GotFocus()Lbl_ErrorMsg.Visible = FalseEnd Sub
The Output Window
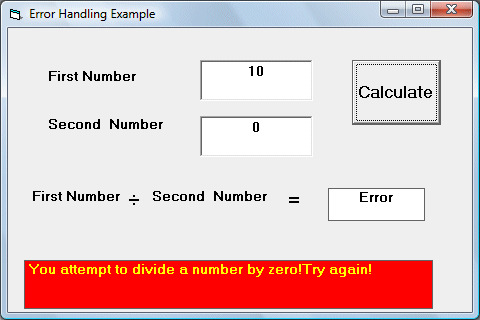
Explanation:
In this example, you design the interface as above. Name the first textbox as Txt_FirstNumber and the second textbox as Txt_SecondNumber. Insert one command button as label it as Calculate. Insert one label and name it as Lbl_Answer to display the answers. If the user enter 0 in the second textbox as shown above, the program will jump to the label error_handler, and the procedure that is executed. It will show an error in the Txt_Answer label and an error message in the Lbl_ErrorMsg label.
Notice that Exit sub after the division. It is important because it can prevent the program to execute the error_handler code even though the user does not enter zero in the second textbox.
Lastly, after the error message appeared, the user will click on the textboxes again. When this occur, the error message will disappear both from the answer label and error message label. This is achieved by using the event procedure GotFocus, as shown in the code.
Example 35.2: Nested Error Handling Procedure
By referring to Example 28.1, we need to consider other errors probably will be made by the user, such as entering non-numeric inputs like letters. Therefore, we need to write error handling code for this error too. It should be put in the first place as soon as the user input something in the textboxes. And the error handler label error_handler1 for this error should be put after the error_handler2 label. This means the second error handling procedure is nested within the first error handling procedure. Notice that you have to put an Exit Sub for the second error handling procedure to prevent to execute the first error handling procedure again. The code is as follow:
Private Sub CmdCalculate_Click()
Dim firstNum, secondNum As Double
On Error GoTo error_handler1
firstNum = Txt_FirstNumber.Text
secondNum = Txt_SecondNumber.Text
On Error GoTo error_handler2
Lbl_Answer.Caption = firstNum / secondNum
Exit Sub 'To prevent errror handling even the inputs are valid
error_handler2:
Lbl_Answer.Caption = "Error"
Lbl_ErrorMsg.Visible = True
Lbl_ErrorMsg.Caption = " You attempt to divide a number by zero!Try again!"
Exit Sub
error_handler1:
Lbl_Answer.Caption = "Error"
Lbl_ErrorMsg.Visible = True
Lbl_ErrorMsg.Caption = " You are not entering a number! Try again!"
End Sub
Private Sub Txt_FirstNumber_GotFocus()
Private Sub Txt_FirstNumber_GotFocus()
Lbl_ErrorMsg.Visible = False
End Sub
Private Sub Txt_SecondNumber_GotFocus()
End SubLbl_ErrorMsg.Visible = False
The Output window
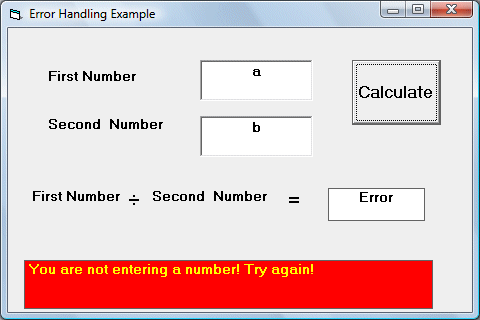
http://www.vbtutor.net/vb6/vbtutor.html
No comments:
Post a Comment